Click Once to Unfocus Textarea Click Again to Submit
Affiliate fifteenTreatment Events
You take ability over your heed—not outside events. Realize this, and y'all volition notice strength.
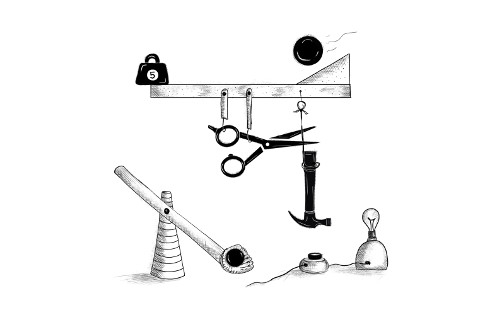
Some programs work with direct user input, such as mouse and keyboard actions. That kind of input isn't available as a well-organized information construction—it comes in slice by slice, in real time, and the program is expected to answer to it as it happens.
Consequence handlers
Imagine an interface where the just manner to observe out whether a cardinal on the keyboard is existence pressed is to read the current state of that central. To be able to react to keypresses, you would have to constantly read the central'southward state and so that yous'd catch it before information technology's released again. It would be dangerous to perform other time-intensive computations since you might miss a keypress.
Some primitive machines practise handle input similar that. A step up from this would be for the hardware or operating organization to notice the keypress and put information technology in a queue. A programme can then periodically check the queue for new events and react to what it finds there.
Of grade, it has to recollect to await at the queue, and to practise it often, because any fourth dimension between the primal being pressed and the program noticing the upshot will cause the software to feel unresponsive. This approach is called polling. Most programmers adopt to avoid it.
A better mechanism is for the arrangement to actively notify our lawmaking when an issue occurs. Browsers do this by assuasive the states to register functions as handlers for specific events.
< p >Click this certificate to actuate the handler.</ p > < script > window.addEventListener("click", () => { console.log("You knocked?"); }); </ script >
The window
binding refers to a built-in object provided past the browser. It represents the browser window that contains the certificate. Calling its addEventListener
method registers the second argument to exist called whenever the event described by its beginning statement occurs.
Events and DOM nodes
Each browser event handler is registered in a context. In the previous example we called addEventListener
on the window
object to register a handler for the whole window. Such a method can besides be found on DOM elements and some other types of objects. Event listeners are called only when the event happens in the context of the object they are registered on.
< button >Click me</ button > < p >No handler hither.</ p > < script > let button = certificate.querySelector("push"); push.addEventListener("click", () => { console.log("Button clicked."); }); </ script >
That case attaches a handler to the button node. Clicks on the button cause that handler to run, but clicks on the rest of the document do non.
Giving a node an onclick
attribute has a similar effect. This works for most types of events—you lot can attach a handler through the attribute whose name is the issue name with on
in front of it.
But a node tin take but 1 onclick
attribute, and then you can annals simply one handler per node that way. The addEventListener
method allows y'all to add together any number of handlers and then that information technology is safe to add handlers even if there is already some other handler on the element.
The removeEventListener
method, called with arguments like to addEventListener
, removes a handler.
< button >Act-once push</ button > < script > allow button = document.querySelector("button"); role one time() { console.log("Done."); button.removeEventListener("click", one time); } push.addEventListener("click", once); </ script >
The part given to removeEventListener
has to exist the same function value that was given to addEventListener
. And so, to unregister a handler, you'll desire to requite the function a name (once
, in the example) to be able to pass the same part value to both methods.
Event objects
Though we have ignored it so far, event handler functions are passed an argument: the event object. This object holds additional information about the issue. For example, if we want to know which mouse push button was pressed, we can look at the event object's push
holding.
< push >Click me any way you desire</ button > < script > let push = certificate.querySelector("push button"); button.addEventListener("mousedown", event => { if (issue.button == 0) { console.log("Left push button"); } else if (event.push == i) { console.log("Centre push"); } else if (event.push == 2) { console.log("Right button"); } }); </ script >
The data stored in an upshot object differs per type of event. We'll discuss unlike types subsequently in the chapter. The object'due south type
holding ever holds a cord identifying the issue (such equally "click"
or "mousedown"
).
Propagation
For most issue types, handlers registered on nodes with children will also receive events that happen in the children. If a button inside a paragraph is clicked, issue handlers on the paragraph will also see the click event.
Just if both the paragraph and the button have a handler, the more specific handler—the one on the push button—gets to get get-go. The event is said to propagate outward, from the node where it happened to that node's parent node and on to the root of the document. Finally, after all handlers registered on a specific node have had their plow, handlers registered on the whole window get a take a chance to respond to the effect.
At whatever point, an event handler can call the stopPropagation
method on the event object to forestall handlers farther up from receiving the event. This can exist useful when, for example, yous accept a button within another clickable element and you lot don't want clicks on the button to activate the outer element'south click beliefs.
The following example registers "mousedown"
handlers on both a button and the paragraph around it. When clicked with the right mouse push button, the handler for the push calls stopPropagation
, which volition prevent the handler on the paragraph from running. When the push button is clicked with some other mouse button, both handlers will run.
< p >A paragraph with a < button >button</ button >.</ p > < script > let para = document.querySelector("p"); permit push button = document.querySelector("button"); para.addEventListener("mousedown", () => { console.log("Handler for paragraph."); }); push button.addEventListener("mousedown", event => { console.log("Handler for push button."); if (event.button == two) event.stopPropagation(); }); </ script >
Most issue objects have a target
property that refers to the node where they originated. You tin can use this property to ensure that you're non accidentally treatment something that propagated up from a node you do non want to handle.
Information technology is as well possible to use the target
property to bandage a wide net for a specific type of event. For example, if you take a node containing a long listing of buttons, information technology may be more than convenient to register a single click handler on the outer node and have it use the target
property to effigy out whether a button was clicked, rather than register individual handlers on all of the buttons.
< button >A</ button > < push >B</ button > < button >C</ button > < script > certificate.body.addEventListener("click", event => { if (event.target.nodeName == "Push") { console.log("Clicked", consequence.target.textContent); } }); </ script >
Default actions
Many events have a default action associated with them. If you click a link, you will be taken to the link'due south target. If you press the down arrow, the browser will scroll the page down. If yous right-click, you'll become a context menu. And and so on.
For most types of events, the JavaScript outcome handlers are called before the default beliefs takes place. If the handler doesn't want this normal behavior to happen, typically because it has already taken care of handling the consequence, it can call the preventDefault
method on the event object.
This can be used to implement your own keyboard shortcuts or context bill of fare. It can also be used to obnoxiously interfere with the behavior that users expect. For example, here is a link that cannot exist followed:
< a href="https://developer.mozilla.org/" >MDN</ a > < script > let link = document.querySelector("a"); link.addEventListener("click", event => { panel.log("Nope."); event.preventDefault(); }); </ script >
Attempt not to practice such things unless yous take a really practiced reason to. It'll be unpleasant for people who utilise your page when expected behavior is broken.
Depending on the browser, some events can't be intercepted at all. On Chrome, for example, the keyboard shortcut to close the current tab (control-West or command-W) cannot be handled past JavaScript.
Key events
When a cardinal on the keyboard is pressed, your browser fires a "keydown"
result. When it is released, you get a "keyup"
upshot.
< p >This page turns violet when yous hold the 5 fundamental.</ p > < script > window.addEventListener("keydown", event => { if (event.key == "v") { document.trunk.mode.background = "violet"; } }); window.addEventListener("keyup", event => { if (event.central == "v") { certificate.body.style.background = ""; } }); </ script >
Despite its proper name, "keydown"
fires non just when the key is physically pushed down. When a key is pressed and held, the event fires over again every time the key repeats. Sometimes you take to exist careful well-nigh this. For instance, if you add a button to the DOM when a cardinal is pressed and remove it again when the key is released, you might accidentally add hundreds of buttons when the central is held downward longer.
The example looked at the primal
holding of the outcome object to see which primal the event is about. This property holds a string that, for most keys, corresponds to the thing that pressing that fundamental would type. For special keys such every bit enter, it holds a cord that names the key ("Enter"
, in this instance). If y'all agree shift while pressing a central, that might likewise influence the proper noun of the fundamental—"v"
becomes "V"
, and "1"
may get "!"
, if that is what pressing shift-one produces on your keyboard.
Modifier keys such as shift, command, alt, and meta (control on Mac) generate key events just like normal keys. But when looking for key combinations, you can also find out whether these keys are held down by looking at the shiftKey
, ctrlKey
, altKey
, and metaKey
properties of keyboard and mouse events.
< p >Printing Command-Space to go along.</ p > < script > window.addEventListener("keydown", event => { if (outcome.key == " " & & event.ctrlKey) { console.log("Standing!"); } }); </ script >
The DOM node where a key event originates depends on the chemical element that has focus when the key is pressed. Most nodes cannot have focus unless you give them a tabindex
attribute, but things similar links, buttons, and grade fields tin can. We'll come up back to form fields in Affiliate 18. When nix in particular has focus, document.body
acts equally the target node of fundamental events.
When the user is typing text, using primal events to effigy out what is beingness typed is problematic. Some platforms, most notably the virtual keyboard on Android phones, don't fire key events. But even when you accept an old-fashioned keyboard, some types of text input don't match central presses in a straightforward way, such every bit input method editor (IME) software used by people whose scripts don't fit on a keyboard, where multiple key strokes are combined to create characters.
To notice when something was typed, elements that you can type into, such every bit the <input>
and <textarea>
tags, burn down "input"
events whenever the user changes their content. To go the bodily content that was typed, it is best to straight read information technology from the focused field. Affiliate xviii will show how.
Arrow events
At that place are currently two widely used ways to signal at things on a screen: mice (including devices that act like mice, such as touchpads and trackballs) and touchscreens. These produce different kinds of events.
Mouse clicks
Pressing a mouse push causes a number of events to fire. The "mousedown"
and "mouseup"
events are like to "keydown"
and "keyup"
and fire when the button is pressed and released. These happen on the DOM nodes that are immediately below the mouse pointer when the outcome occurs.
Afterward the "mouseup"
result, a "click"
effect fires on the most specific node that contained both the press and the release of the button. For case, if I press down the mouse push button on one paragraph and then move the pointer to another paragraph and release the button, the "click"
outcome volition happen on the element that contains both those paragraphs.
If two clicks happen close together, a "dblclick"
(double-click) consequence also fires, afterward the second click event.
To get precise information almost the place where a mouse event happened, y'all can look at its clientX
and clientY
backdrop, which contain the event'south coordinates (in pixels) relative to the top-left corner of the window, or pageX
and pageY
, which are relative to the top-left corner of the whole document (which may be different when the window has been scrolled).
The following implements a archaic cartoon programme. Every time you click the document, it adds a dot under your mouse arrow. See Affiliate 19 for a less archaic drawing plan.
< style > trunk { peak: 200px; background: beige; } .dot { height: 8px; width: 8px; edge-radius: 4px; background: blue; position: absolute; } </ fashion > < script > window.addEventListener("click", event => { let dot = document.createElement("div"); dot.className = "dot"; dot.manner.left = (event.pageX - iv) + "px"; dot.manner.top = (event.pageY - 4) + "px"; document.body.appendChild(dot); }); </ script >
Mouse motion
Every time the mouse pointer moves, a "mousemove"
event is fired. This outcome tin be used to runway the position of the mouse. A common situation in which this is useful is when implementing some form of mouse-dragging functionality.
As an instance, the following program displays a bar and sets up event handlers so that dragging to the left or right on this bar makes it narrower or wider:
< p >Drag the bar to alter its width:</ p > < div style="background: orange; width: 60px; pinnacle: 20px" > </ div > < script > allow lastX; let bar = document.querySelector("div"); bar.addEventListener("mousedown", event => { if (event.push == 0) { lastX = issue.clientX; window.addEventListener("mousemove", moved); event.preventDefault(); } }); function moved(event) { if (upshot.buttons == 0) { window.removeEventListener("mousemove", moved); } else { let dist = event.clientX - lastX; allow newWidth = Math.max(10, bar.offsetWidth + dist); bar.style.width = newWidth + "px"; lastX = outcome.clientX; } } </ script >
Note that the "mousemove"
handler is registered on the whole window. Even if the mouse goes outside of the bar during resizing, as long as the button is held we still want to update its size.
We must stop resizing the bar when the mouse button is released. For that, nosotros can use the buttons
property (annotation the plural), which tells us virtually the buttons that are currently held down. When this is naught, no buttons are down. When buttons are held, its value is the sum of the codes for those buttons—the left button has code 1, the right button two, and the middle 1 4. With the left and correct buttons held, for example, the value of buttons
will exist three.
Notation that the club of these codes is unlike from the 1 used by button
, where the eye push came before the right 1. Every bit mentioned, consistency isn't really a strong point of the browser's programming interface.
Touch events
The style of graphical browser that nosotros use was designed with mouse interfaces in mind, at a time where touchscreens were rare. To make the Web "piece of work" on early touchscreen phones, browsers for those devices pretended, to a certain extent, that touch events were mouse events. If you tap your screen, y'all'll go "mousedown"
, "mouseup"
, and "click"
events.
But this illusion isn't very robust. A touchscreen works differently from a mouse: information technology doesn't accept multiple buttons, yous tin't track the finger when it isn't on the screen (to simulate "mousemove"
), and it allows multiple fingers to be on the screen at the same time.
Mouse events embrace touch interaction only in straightforward cases—if you add a "click"
handler to a button, affect users will still exist able to use it. But something similar the resizeable bar in the previous example does not work on a touchscreen.
In that location are specific outcome types fired past bear on interaction. When a finger starts touching the screen, you go a "touchstart"
event. When it is moved while touching, "touchmove"
events fire. Finally, when information technology stops touching the screen, you'll see a "touchend"
outcome.
Considering many touchscreens can find multiple fingers at the same time, these events don't accept a single set of coordinates associated with them. Rather, their effect objects take a touches
holding, which holds an assortment-like object of points, each of which has its own clientX
, clientY
, pageX
, and pageY
backdrop.
You could do something like this to evidence red circles around every touching finger:
< fashion > dot { position: accented; display: cake; edge: 2px solid ruddy; border-radius: 50px; height: 100px; width: 100px; } </ style > < p >Touch this page</ p > < script > office update(issue) { for (allow dot; dot = document.querySelector("dot");) { dot.remove(); } for (let i = 0; i < event.touches.length; i ++) { allow {pageX, pageY} = event.touches[i]; allow dot = document.createElement("dot"); dot.style.left = (pageX - 50) + "px"; dot.manner.height = (pageY - 50) + "px"; document.body.appendChild(dot); } } window.addEventListener("touchstart", update); window.addEventListener("touchmove", update); window.addEventListener("touchend", update); </ script >
You'll often want to call preventDefault
in touch event handlers to override the browser's default behavior (which may include scrolling the page on swiping) and to forbid the mouse events from being fired, for which yous may also have a handler.
Ringlet events
Whenever an element is scrolled, a "coil"
event is fired on it. This has various uses, such as knowing what the user is currently looking at (for disabling off-screen animations or sending spy reports to your evil headquarters) or showing some indication of progress (by highlighting function of a table of contents or showing a folio number).
The following instance draws a progress bar above the document and updates information technology to fill upward as you scroll down:
< style > #progress { border-bottom: 2px solid bluish; width: 0; position: fixed; top: 0; left: 0; } </ mode > < div id="progress" > </ div > < script > certificate.trunk.appendChild(certificate.createTextNode( "supercalifragilisticexpialidocious ".repeat(k))); let bar = document.querySelector("#progress"); window.addEventListener("roll", () => { allow max = document.body.scrollHeight - innerHeight; bar.style.width = `${(pageYOffset / max) * 100 } %`; }); </ script >
Giving an chemical element a position
of stock-still
acts much like an absolute
position but also prevents it from scrolling along with the rest of the certificate. The effect is to make our progress bar stay at the summit. Its width is inverse to indicate the current progress. We use %
, rather than px
, every bit a unit when setting the width and so that the element is sized relative to the page width.
The global innerHeight
binding gives us the tiptop of the window, which we accept to subtract from the full scrollable height—you lot can't keep scrolling when you hitting the bottom of the document. There's likewise an innerWidth
for the window width. By dividing pageYOffset
, the current gyre position, by the maximum curl position and multiplying by 100, we get the percent for the progress bar.
Calling preventDefault
on a curlicue event does non preclude the scrolling from happening. In fact, the upshot handler is chosen only later on the scrolling takes place.
Focus events
When an element gains focus, the browser fires a "focus"
event on it. When information technology loses focus, the element gets a "blur"
event.
Unlike the events discussed earlier, these 2 events practise not propagate. A handler on a parent element is not notified when a child chemical element gains or loses focus.
The post-obit case displays assistance text for the text field that currently has focus:
< p >Name: < input type="text" information-help="Your full name" > </ p > < p >Age: < input blazon="text" data-help="Your age in years" > </ p > < p id="help" > </ p > < script > let help = document.querySelector("#assistance"); let fields = document.querySelectorAll("input"); for (let field of Array.from(fields)) { field.addEventListener("focus", event => { let text = event.target.getAttribute("data-help"); help.textContent = text; }); field.addEventListener("mistiness", outcome => { help.textContent = ""; }); } </ script >
The window object will receive "focus"
and "mistiness"
events when the user moves from or to the browser tab or window in which the document is shown.
Load event
When a folio finishes loading, the "load"
event fires on the window and the document body objects. This is oftentimes used to schedule initialization actions that require the whole document to take been built. Retrieve that the content of <script>
tags is run immediately when the tag is encountered. This may be too shortly, for example when the script needs to do something with parts of the document that announced after the <script>
tag.
Elements such every bit images and script tags that load an external file also take a "load"
event that indicates the files they reference were loaded. Similar the focus-related events, loading events do not propagate.
When a page is closed or navigated away from (for example, by post-obit a link), a "beforeunload"
outcome fires. The principal use of this event is to preclude the user from accidentally losing piece of work by closing a certificate. If you lot prevent the default behavior on this event and prepare the returnValue
property on the event object to a string, the browser will show the user a dialog asking if they actually desire to leave the folio. That dialog might include your string, but because some malicious sites try to employ these dialogs to confuse people into staying on their page to look at dodgy weight loss ads, most browsers no longer display them.
Events and the effect loop
In the context of the upshot loop, as discussed in Chapter 11, browser event handlers acquit like other asynchronous notifications. They are scheduled when the event occurs merely must wait for other scripts that are running to finish before they get a adventure to run.
The fact that events can be processed merely when nothing else is running means that, if the issue loop is tied upwards with other work, any interaction with the page (which happens through events) will be delayed until there'due south time to process it. So if y'all schedule too much piece of work, either with long-running outcome handlers or with lots of short-running ones, the folio volition become slow and cumbersome to use.
For cases where you lot really do want to do some time-consuming affair in the background without freezing the page, browsers provide something called web workers. A worker is a JavaScript process that runs alongside the main script, on its ain timeline.
Imagine that squaring a number is a heavy, long-running ciphering that we want to perform in a separate thread. We could write a file called lawmaking/
that responds to messages past calculating a square and sending a bulletin back.
addEventListener("message", event => { postMessage(result.information * issue.data); });
To avoid the problems of having multiple threads touching the same data, workers do not share their global scope or any other data with the main script's surround. Instead, you lot have to communicate with them past sending messages back and forth.
This lawmaking spawns a worker running that script, sends it a few letters, and outputs the responses.
allow squareWorker = new Worker("code/squareworker.js"); squareWorker.addEventListener("message", effect => { console.log("The worker responded:", event.data); }); squareWorker.postMessage(10); squareWorker.postMessage(24);
The postMessage
function sends a bulletin, which will cause a "message"
effect to fire in the receiver. The script that created the worker sends and receives letters through the Worker
object, whereas the worker talks to the script that created it past sending and listening straight on its global scope. Only values that tin be represented as JSON tin be sent as messages—the other side volition receive a copy of them, rather than the value itself.
Timers
We saw the setTimeout
function in Affiliate 11. Information technology schedules another function to be called later, after a given number of milliseconds.
Sometimes you need to cancel a role y'all have scheduled. This is done by storing the value returned past setTimeout
and calling clearTimeout
on it.
allow bombTimer = setTimeout(() => { console.log("Boom!"); }, 500); if (Math.random() < 0.5) { console.log("Defused."); clearTimeout(bombTimer); }
The cancelAnimationFrame
function works in the aforementioned manner as clearTimeout
—calling it on a value returned by requestAnimationFrame
will abolish that frame (assuming it hasn't already been called).
A similar gear up of functions, setInterval
and clearInterval
, are used to set up timers that should repeat every X milliseconds.
let ticks = 0; permit clock = setInterval(() => { panel.log("tick", ticks ++); if (ticks == ten) { clearInterval(clock); console.log("stop."); } }, 200);
Debouncing
Some types of events have the potential to fire rapidly, many times in a row (the "mousemove"
and "scroll"
events, for example). When handling such events, you lot must be careful not to do anything too time-consuming or your handler will have upwards so much time that interaction with the certificate starts to feel boring.
If yous do demand to exercise something nontrivial in such a handler, you lot tin can use setTimeout
to make sure yous are not doing it too often. This is usually called debouncing the event. There are several slightly different approaches to this.
In the kickoff case, we want to react when the user has typed something, but we don't desire to do information technology immediately for every input effect. When they are typing apace, we but want to wait until a pause occurs. Instead of immediately performing an activeness in the issue handler, nosotros set a timeout. Nosotros likewise clear the previous timeout (if any) so that when events occur close together (closer than our timeout delay), the timeout from the previous event volition be canceled.
< textarea >Type something hither...</ textarea > < script > let textarea = document.querySelector("textarea"); allow timeout; textarea.addEventListener("input", () => { clearTimeout(timeout); timeout = setTimeout(() => console.log("Typed!"), 500); }); </ script >
Giving an undefined value to clearTimeout
or calling it on a timeout that has already fired has no effect. Thus, nosotros don't have to be careful about when to call information technology, and we simply practise then for every event.
We can utilize a slightly unlike pattern if we want to space responses so that they're separated by at least a sure length of time but want to fire them during a series of events, not just afterward. For example, we might desire to answer to "mousemove"
events by showing the current coordinates of the mouse merely but every 250 milliseconds.
< script > permit scheduled = aught; window.addEventListener("mousemove", event => { if (! scheduled) { setTimeout(() => { document.body.textContent = `Mouse at ${ scheduled.pageX } , ${ scheduled.pageY } `; scheduled = null; }, 250); } scheduled = event; }); </ script >
Summary
Event handlers make it possible to detect and react to events happening in our web page. The addEventListener
method is used to register such a handler.
Each event has a blazon ("keydown"
, "focus"
, and so on) that identifies it. Near events are chosen on a specific DOM chemical element and so propagate to that chemical element's ancestors, allowing handlers associated with those elements to handle them.
When an event handler is called, it is passed an consequence object with additional data about the result. This object also has methods that allow us to stop further propagation (stopPropagation
) and prevent the browser'southward default handling of the issue (preventDefault
).
Pressing a central fires "keydown"
and "keyup"
events. Pressing a mouse push fires "mousedown"
, "mouseup"
, and "click"
events. Moving the mouse fires "mousemove"
events. Touchscreen interaction will event in "touchstart"
, "touchmove"
, and "touchend"
events.
Scrolling can be detected with the "curlicue"
issue, and focus changes can be detected with the "focus"
and "blur"
events. When the document finishes loading, a "load"
issue fires on the window.
Exercises
Balloon
Write a page that displays a balloon (using the airship emoji, 🎈). When yous press the up arrow, it should inflate (grow) x percentage, and when you lot printing the down arrow, information technology should deflate (shrink) x percent.
Y'all tin can control the size of text (emoji are text) by setting the font-size
CSS property (style.fontSize
) on its parent element. Remember to include a unit of measurement in the value—for example, pixels (10px
).
The key names of the arrow keys are "ArrowUp"
and "ArrowDown"
. Brand sure the keys change but the balloon, without scrolling the page.
When that works, add a characteristic where, if you blow up the balloon past a certain size, it explodes. In this case, exploding means that it is replaced with an 💥 emoji, and the event handler is removed (so that you can't inflate or deflate the explosion).
< p >🎈</ p > < script > </ script >
You'll want to register a handler for the "keydown"
event and look at event.cardinal
to figure out whether the up or downward arrow primal was pressed.
The current size can be kept in a binding and then that you lot can base the new size on it. It'll be helpful to define a office that updates the size—both the binding and the style of the balloon in the DOM—so that you tin can call it from your event handler, and possibly also once when starting, to set the initial size.
You can change the balloon to an explosion past replacing the text node with another one (using replaceChild
) or by setting the textContent
property of its parent node to a new string.
Mouse trail
In JavaScript's early days, which was the loftier time of gaudy abode pages with lots of animated images, people came upward with some truly inspiring ways to use the language.
I of these was the mouse trail—a series of elements that would follow the mouse pointer equally yous moved it across the page.
In this exercise, I desire yous to implement a mouse trail. Utilize absolutely positioned <div>
elements with a stock-still size and groundwork color (refer to the lawmaking in the "Mouse Clicks" section for an case). Create a bunch of such elements and, when the mouse moves, brandish them in the wake of the mouse pointer.
There are various possible approaches here. You lot tin can make your solution as simple or as circuitous as you want. A elementary solution to outset with is to keep a fixed number of trail elements and cycle through them, moving the next 1 to the mouse's current position every time a "mousemove"
result occurs.
< manner > .trail { position: absolute; height: 6px; width: 6px; border-radius: 3px; background: teal; } body { pinnacle: 300px; } </ style > < script > </ script >
Creating the elements is best done with a loop. Suspend them to the certificate to brand them evidence up. To exist able to access them later to alter their position, you'll want to store the elements in an array.
Cycling through them can be done by keeping a counter variable and adding 1 to it every fourth dimension the "mousemove"
upshot fires. The remainder operator (% elements.
) can then be used to become a valid array index to pick the chemical element you desire to position during a given effect.
Another interesting event can be achieved by modeling a uncomplicated physics system. Use the "mousemove"
event just to update a pair of bindings that track the mouse position. And then use requestAnimationFrame
to simulate the trailing elements beingness attracted to the position of the mouse pointer. At every blitheness step, update their position based on their position relative to the pointer (and, optionally, a speed that is stored for each element). Figuring out a adept fashion to practice this is up to you.
Tabs
Tabbed panels are widely used in user interfaces. They permit you to select an interface panel by choosing from a number of tabs "sticking out" above an element.
In this do you lot must implement a simple tabbed interface. Write a office, asTabs
, that takes a DOM node and creates a tabbed interface showing the child elements of that node. It should insert a list of <button>
elements at the superlative of the node, one for each child chemical element, containing text retrieved from the data-tabname
attribute of the child. All but one of the original children should be hidden (given a display
style of none
). The currently visible node can be selected by clicking the buttons.
When that works, extend it to manner the button for the currently selected tab differently so that information technology is obvious which tab is selected.
< tab-panel > < div data-tabname="1" >Tab one</ div > < div information-tabname="two" >Tab two</ div > < div data-tabname="three" >Tab 3</ div > </ tab-panel > < script > office asTabs(node) { } asTabs(document.querySelector("tab-panel")); </ script >
I pitfall y'all might come across is that you can't directly use the node's childNodes
property as a drove of tab nodes. For one affair, when you add the buttons, they will also get child nodes and stop up in this object because information technology is a live data structure. For some other, the text nodes created for the whitespace betwixt the nodes are also in childNodes
but should not go their own tabs. Yous can utilize children
instead of childNodes
to ignore text nodes.
You could commencement by building upward an array of tabs so that yous accept easy access to them. To implement the styling of the buttons, yous could store objects that incorporate both the tab console and its button.
I recommend writing a split function for changing tabs. You lot tin can either shop the previously selected tab and change merely the styles needed to hide that and show the new one, or you can just update the style of all tabs every time a new tab is selected.
You lot might want to call this function immediately to brand the interface start with the first tab visible.
Source: https://eloquentjavascript.net/15_event.html
0 Response to "Click Once to Unfocus Textarea Click Again to Submit"
แสดงความคิดเห็น